Key Value Storage
NuxtHub Key Value Storage uses Unstorage with Cloudflare Workers KV to store key-value data.
Use Cases
- Frequently Read Data - values are cached in regional data centers closer to the user so multiple requests from the same region will be faster
- Per-Object Expiration - passing a
ttl
when writing object will delete it after a certain amount of time - Eventual Consistency - cached values are eventually consistent and may take up to 60 seconds to update across all regions, allowing for improved performance when strong consistency is not required
Getting Started
Enable the key-value storage in your NuxtHub project by adding the kv
property to the hub
object in your nuxt.config.ts
file.
export default defineNuxtConfig({
hub: {
kv: true
}
})
You can inspect your KV namespace during local development in the Nuxt DevTools or after a deployment using the NuxtHub Admin Dashboard.
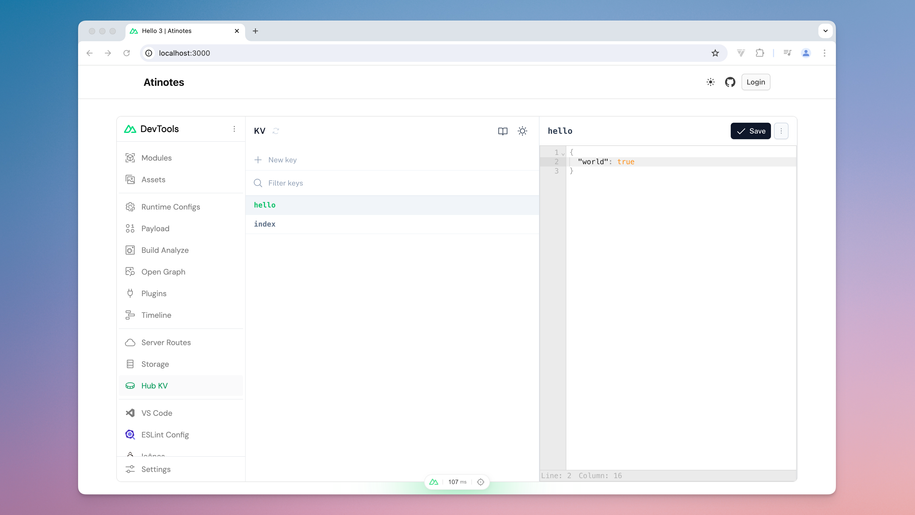
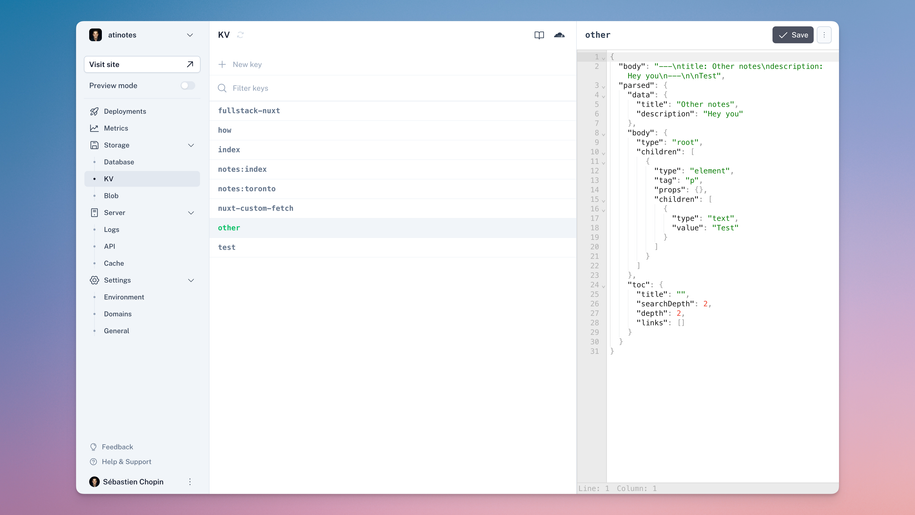
How KV Works
NuxtHub uses Cloudflare Workers KV to store key-value data a few centralized data centers. Then, when data is requested, it will cache the responses in regional data centers closer to the user to speed up future requests coming from the same region.
This caching means that KV is optimized for high-read use cases, but it also means that changes like editing or deleting data are eventually consistent and may take up to 60 seconds to propagate to all regions. Even if a request is made for a key that does not exist in the KV namespace, that result will be cached for up to 60 seconds.
If you need a strongly consistent data model, where changes are immediately visible to all users, a NuxtHub database may be a better fit.
To learn more about how KV works, check out the Cloudflare KV documentation.
hubKV()
hubKV()
is a server composable that returns an Unstorage instance with Cloudflare KV binding as the driver.
Set an item
Puts an item in the storage.
await hubKV().set('vue', { year: 2014 })
// using prefixes to organize your KV namespace, useful for the `keys` operation
await hubKV().set('vue:nuxt', { year: 2016 })
Expiration
By default, items in your KV namespace will never expire. You can delete them manually using the del()
method or set a TTL (time to live) in seconds.
The item will be deleted after the TTL has expired. The ttl
option maps to Cloudflare's expirationTtl
option. Values that have recently been read will continue to return the cached value for up to 60 seconds and may not be immediately deleted for all regions.
await hubKV().set('vue:nuxt', { year: 2016 }, { ttl: 60 })
Get an item
Retrieves an item from the Key-Value storage.
const vue = await hubKV().get('vue')
/*
{
year: 2014
}
*/
Has an item
Checks if an item exists in the storage.
const hasAngular = await hubKV().has('angular') // false
const hasVue = await hubKV().has('vue') // true
Delete an item
Delete an item with the given key from the storage.
await hubKV().del('react')
Clear the KV namespace
Deletes all items from the KV namespace..
await hubKV().clear()
To delete all items for a specific prefix, you can pass the prefix as an argument. We recommend using prefixes for better organization in your KV namespace.
await hubKV().clear('react')
List all keys
Retrieves all keys from the KV storage.
const keys = await hubKV().keys()
/*
[
'react',
'react:gatsby',
'react:next',
'vue',
'vue:nuxt',
'vue:quasar'
]
To get the keys starting with a specific prefix, you can pass the prefix as an argument. We recommend using prefixes for better organization in your KV namespace.
const vueKeys = await hubKV().keys('vue')
/*
[
'vue:nuxt',
'vue:quasar'
]
*/
Limits
- The maximum size of a value is 25 MiB.
- The maximum length of a key is 512 bytes.
- The TTL must be at least 60 seconds.
- There is a maximum of 1 write to the same key per second (KV write rate limit).
Learn more about Cloudflare KV limits.
Learn More
hubKV()
is an instance of unstorage with the Cloudflare KV binding driver.
Pricing
Free | Workers Paid ($5/month) | |
---|---|---|
Read | 100,000 / day | 10 million / month + $0.50/million |
List | 1,000 / day | 1 million / month + $5.00/million |
Write | 1,000 / day | 1 million / month + $5.00/million |
Delete | 1,000 / day | 1 million / month + $5.00/million |
Storage | 1 GB | 1 GB + $0.50/GB-month |