Cache Pages, API & Functions
Getting Started
Enable the cache storage in your NuxtHub project by adding the cache
property to the hub
object in your nuxt.config.ts
file.
export default defineNuxtConfig({
hub: {
cache: true
}
})
Once your Nuxt project is deployed, you can manage your cache entries in the Cache
section of your project in the NuxtHub admin.
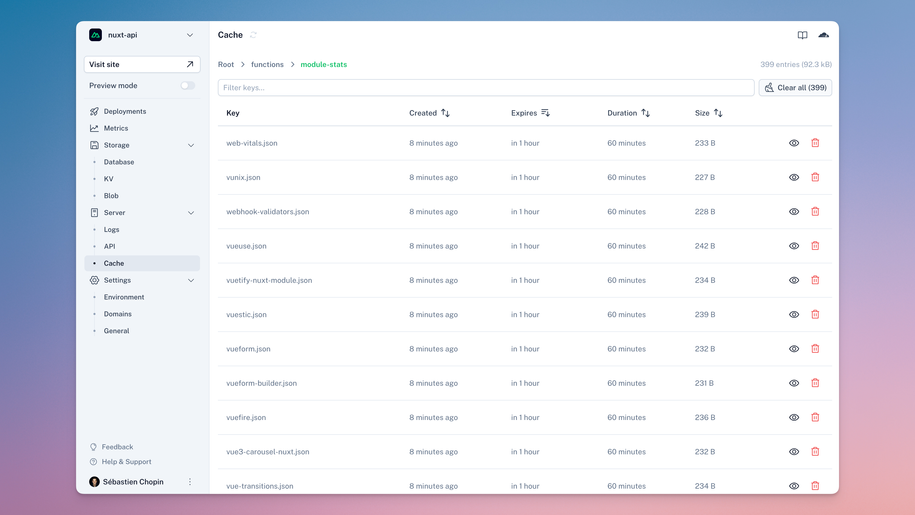
In development, checkout the Hub Cache section in the Nuxt Devtools.
API Routes Caching
To cache Nuxt API and server routes, use the cachedEventHandler
function. This function will cache the response of the server route into the cache storage.
import type { H3Event } from 'h3'
export default cachedEventHandler((event) => {
return {
success: true,
date: new Date().toISOString()
}
}, {
maxAge: 60 * 60, // 1 hour
getKey: (event: H3Event) => event.path
})
The above example will cache the response of the /api/cached-route
route for 1 hour. The getKey
function is used to generate the key for the cache entry.
Server Functions Caching
Using the cachedFunction
function, You can cache the response of a server function based on the arguments passed to the function.
import type { H3Event } from 'h3'
export const getRepoStarCached = defineCachedFunction(async (event: H3Event, repo: string) => {
const data: any = await $fetch(`https://api.github.com/repos/${repo}`)
return data.stargazers_count
}, {
maxAge: 60 * 60, // 1 hour
name: 'ghStars',
getKey: (event: H3Event, repo: string) => repo
})
The above example will cache the result of the getRepoStarCached
function for 1 hour.
event
argument should always be the first argument of the cached function. Nitro leverages event.waitUntil
to keep the instance alive while the cache is being updated while the response is sent to the client.Read more about this in the Nitro docs.
Cache Invalidation
When using the defineCachedFunction
or defineCachedEventHandler
functions, the cache key is generated using the following pattern:
`${options.group}:${options.name}:${options.getKey(...args)}.json`
The defaults are:
group
:'nitro'
name
:'handlers'
for api routes and'functions'
for server functions
For example, the following function:
const getAccessToken = defineCachedFunction(() => {
return String(Date.now())
}, {
maxAge: 10,
name: 'getAccessToken',
getKey: () => 'default'
})
Will generate the following cache key:
nitro:functions:getAccessToken:default.json
You can invalidate the cached function entry with:
await useStorage('cache').removeItem('nitro:functions:getAccessToken:default.json')
Cache Expiration
As NuxtHub leverages Cloudflare Workers KV to store your cache entries, we leverage the expiration
property of the KV binding to handle the cache expiration.
maxAge
) lower than 60
seconds, NuxtHub will set the KV entry expiration to 60
seconds in the future (Cloudflare KV limitation) so it can be removed automatically.